In this example I’ve created a map of pairs int and float and then filled it out with some values. As I am interested only in the first and last value, I will not iterate through the map, I use begin() and advance() instead. Why not the end()? Because it points to the very next value out of range of map.
map<int, float> mif;
map<int, float>::iterator mifi;
mif.emplace(11, 11.1f);
mif.emplace(12, 12.2f);
mif.emplace(13, 13.3f);
mif.emplace(14, 14.4f);
mif.emplace(15, 15.5f);
mif.emplace(16, 16.6f);
mif.emplace(17, 17.7f);
int myFirst;
float mySecond;
cout << "The content of the map<int, float>: " << endl;
for (pair<int, float> mf : mif)
{
myFirst = mf.first;
mySecond = mf.second;
cout << myFirst << "\t";
cout << mySecond << endl;
}
cout << "The first pair:" << endl;
mifi = mif.begin();
cout << mifi->first << "\t";
cout << mifi->second << endl;
cout << "The last pair:" << endl;
//mifi = mif.end();
advance(mifi, mif.size() - 1);
cout << mifi->first << "\t";
cout << mifi->second << endl;
And the result is:
The content of the map:
11 11.1
12 12.2
13 13.3
14 14.4
15 15.5
16 16.6
17 17.7
The first pair:
11 11.1
The last pair:
17 17.7
When mif.end() was used, then debug assertion failed occurs. This is true only when Debug configuration was chosen.
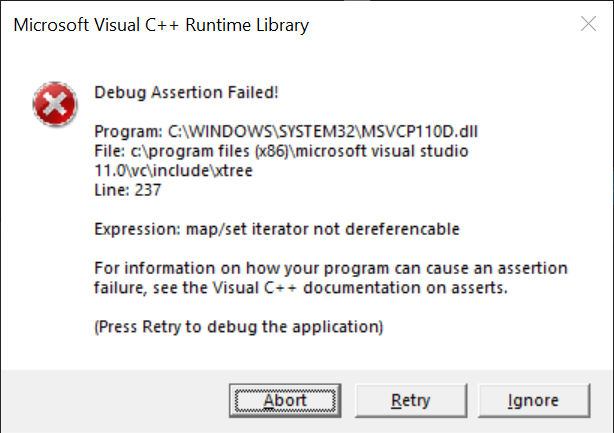
When Release configuration chosen, then no assertion occurs. But the result for the last pair is random:
The first pair:
11 11.1
The last pair:
6619244 8.44903e-039
Now let disassembly this part:
CPU Disasm
Address Hex dump Command Comments
00401B5C |. 68 6B274000 |PUSH 0040276B ; Entry point
00401B61 |. 51 |PUSH ECX
00401B62 |. 8B0D 78504000 |MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401B68 |. F30F110424 |MOVSS DWORD PTR SS:[ESP],XMM0
00401B6D |. FF15 4C504000 |CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<ch
00401B73 |. 8BC8 |MOV ECX,EAX
00401B75 |. FF15 44504000 |CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<ch ; \MSVCP140.std::basic_ostream<unsigned short,std::char_traits<unsigned short> >::operator<<
00401B7B |. 8D4D E4 |LEA ECX,[EBP-1C]
00401B7E |. E8 97020000 |CALL 00401E1A
00401B83 |. 8B45 E4 |MOV EAX,DWORD PTR SS:[EBP-1C]
00401B86 |.^ EB A9 \JMP SHORT 00401B31
00401B88 |> 68 6B274000 PUSH 0040276B ; /Arg1 = ConsoleApplication2.40276B, Entry point
00401B8D |. BA 20544000 MOV EDX,OFFSET 00405420 ; |ASCII "The first pair:"
00401B92 |. E8 100A0000 CALL 004025A7 ; |output "The first pair"
00401B97 |. 8BC8 MOV ECX,EAX ; |
00401B99 |. FF15 44504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; \MSVCP140.std::basic_ostream<unsigned short,std::char_traits<unsigned short> >::operator<<
00401B9F |. 8B75 E8 MOV ESI,DWORD PTR SS:[EBP-18]
00401BA2 |. 8B0D 78504000 MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401BA8 |. 8B36 MOV ESI,DWORD PTR DS:[ESI]
00401BAA |. FF76 10 PUSH DWORD PTR DS:[ESI+10] ; value to output
00401BAD |. FF15 48504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; output first value
00401BB3 |. 8BD7 MOV EDX,EDI
00401BB5 |. 8BC8 MOV ECX,EAX
00401BB7 |. E8 EB090000 CALL 004025A7 ; output TAB
00401BBC |. F30F1046 14 MOVSS XMM0,DWORD PTR DS:[ESI+14] ; float 11.1
00401BC1 |. 68 6B274000 PUSH 0040276B ; Entry point
00401BC6 |. 51 PUSH ECX
00401BC7 |. 8B0D 78504000 MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401BCD |. F30F110424 MOVSS DWORD PTR SS:[ESP],XMM0
00401BD2 |. FF15 4C504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; output 11.1
00401BD8 |. 8BC8 MOV ECX,EAX ; new line
00401BDA |. FF15 44504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; \MSVCP140.std::basic_ostream<unsigned short,std::char_traits<unsigned short> >::operator<<
00401BE0 |. 8B0D 78504000 MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401BE6 |. BA 30544000 MOV EDX,OFFSET 00405430 ; ASCII "The last pair:"
00401BEB |. 68 6B274000 PUSH 0040276B ; /Arg1 = ConsoleApplication2.40276B, Entry point
00401BF0 |. E8 B2090000 CALL 004025A7 ; |output "The last pair"
00401BF5 |. 8BC8 MOV ECX,EAX ; |new line
00401BF7 |. FF15 44504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; \MSVCP140.std::basic_ostream<unsigned short,std::char_traits<unsigned short> >::operator<<
00401BFD |. 8B75 E8 MOV ESI,DWORD PTR SS:[EBP-18]
00401C00 |. 8B0D 78504000 MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401C06 |. FF76 10 PUSH DWORD PTR DS:[ESI+10]
00401C09 |. FF15 48504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; output first random value
00401C0F |. 8BD7 MOV EDX,EDI ; 9
00401C11 |. 8BC8 MOV ECX,EAX
00401C13 |. E8 8F090000 CALL 004025A7 ; output TAB
00401C18 |. F30F1046 14 MOVSS XMM0,DWORD PTR DS:[ESI+14]
00401C1D |. 68 6B274000 PUSH 0040276B ; Entry point
00401C22 |. 51 PUSH ECX
00401C23 |. 8B0D 78504000 MOV ECX,DWORD PTR DS:[<&MSVCP140.std::cout>]
00401C29 |. F30F110424 MOVSS DWORD PTR SS:[ESP],XMM0
00401C2E |. FF15 4C504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; output random float
00401C34 |. 8BC8 MOV ECX,EAX ; new line
00401C36 |. FF15 44504000 CALL DWORD PTR DS:[<&MSVCP140.std::basic_ostream<cha ; \MSVCP140.std::basic_ostream<unsigned short,std::char_traits<unsigned short> >::operator<<
00401C3C |. 8D4D E8 LEA ECX,[EBP-18]
Ten piękny listing pochodzi z OllyDbg poprzez zaznaczenie fragmentu i Control+C (Edit -> Copy as table), a następnie do WP jako blok SyntaxHighlighter type Arduino. Komentarze tez z OllyDbg za pomocą Control-A (Analysis -> analyze code) .